In this tutorial, we will see how to get touch input from a mobile device and use it in your game. We will see how to do this in both the old and new input system of Unity.
Get touch input using Unity’s old Input system
Old input system does not require any setup. It’s inbuild and configured by default in Unity. It all comes down to using it in code.
The first step is to check if any touch is recorded
Input.touchCount
If any touch is recorded it is saved as an array. To get the first touch
Input.GetTouch(0)
If you want to check the finger status on the screen.
TouchPhase.Began
TouchPhase.Moved
TouchPhase.Ended
Here is the complete script
void Update ()
{
if (Input.touchCount > 0)
{
Touch touch = Input.GetTouch(0);
// Move the cube if the screen has the finger moving.
if (touch.phase == TouchPhase.Moved)
{
Debug.Log(touch.position);
}
}
}
If you have a GUI based game then you can use the touch position as shown below
pos.x = (pos.x - width) / width;
pos.y = (pos.y - height) / height;
In case you want to use the touch position in world space then you can convert it using the below line of code.
vector2 world_pos=Camera.main.ScreenToWorldPoint(touch.position);
Get touch input using Unity’s new Input system
New input system requires configuration. Let’s set it up to be able to do all the above tasks we did with the old system.
Import the new input system using Unity package manager.
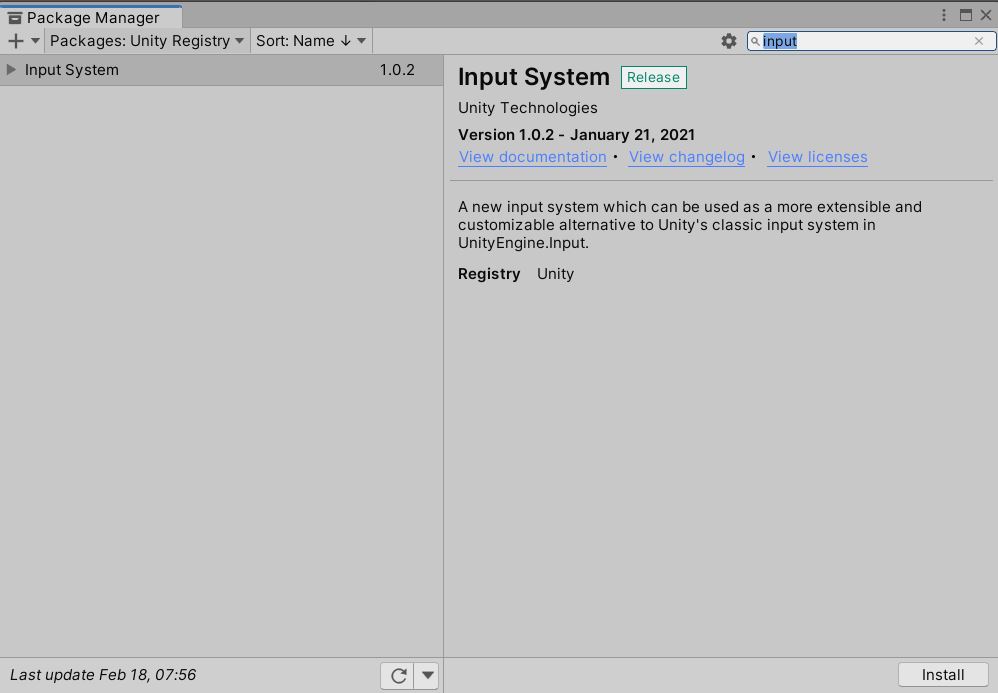
Note: This might reload your project.
We need to create a new script for the new input system. We will be using the enhanced touch API for this tutorial.
You need to add “using UnityEngine.InputSystem.EnhancedTouch” to your script.
You can change the Touch.onFingerDown to the options shown in the image below based on your requirement.
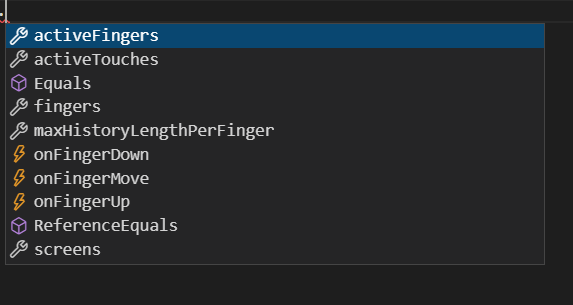
using UnityEngine;
using UnityEngine.InputSystem.EnhancedTouch;
public class get_touch_input : MonoBehaviour
{
void OnEnable()
{
TouchSimulation.Enable();
EnhancedTouchSupport.Enable();
UnityEngine.InputSystem.EnhancedTouch.Touch.onFingerDown += get_touch_details;
}
void get_touch_details(Finger fin)
{
Debug.Log(fin.screenPosition);
}