Moving a player based on keyboard input is the simplest script that is needed for a game. In this tutorial, we will see how to move a player based on keyboard input in Godot in both 2D and 3D. I am using Godot version 3.4.2 for this tutorial.
Mapping the input
Before you can take the keyboard input you need to map the input in Godot. To map the keyboard keys, go to Project>Project settings and select the Input Map tab. It should look something like this
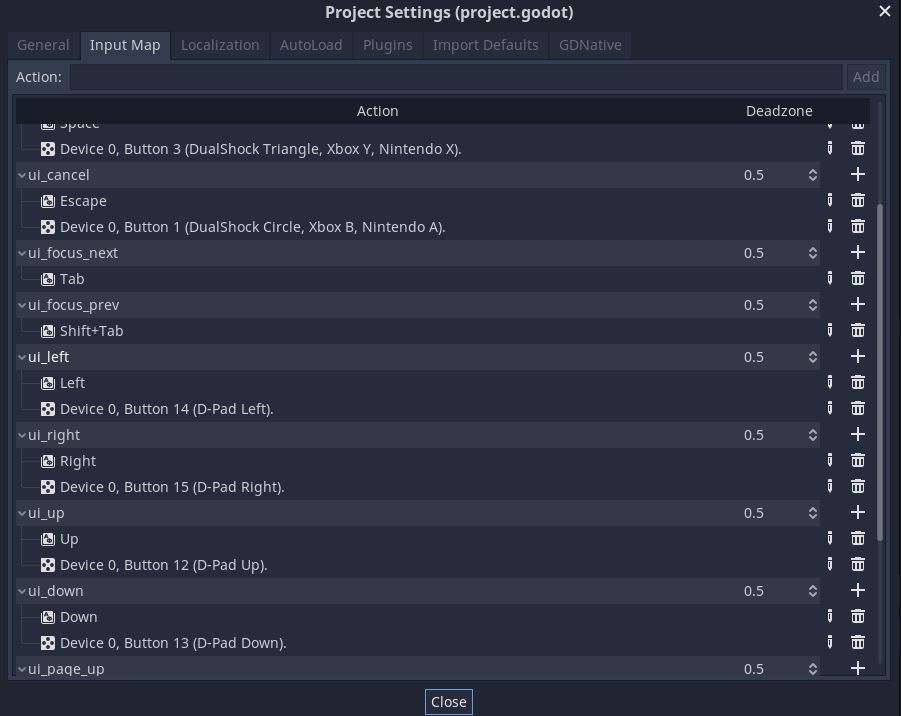
In the newer version of Godot some keys are already set and you can use them directly in your code. If in your version the keys are not mapped then follow the instruction below.
- Type in a new action name and press ADD.
- Press the + sign on the right top corner and select physical key.
- Press the key you want to map.
- Godot displays the key presses on the popup. Click ok to save key.
- Create new action for all directions like Up, Down, Left, right.
Moving a player in 2D
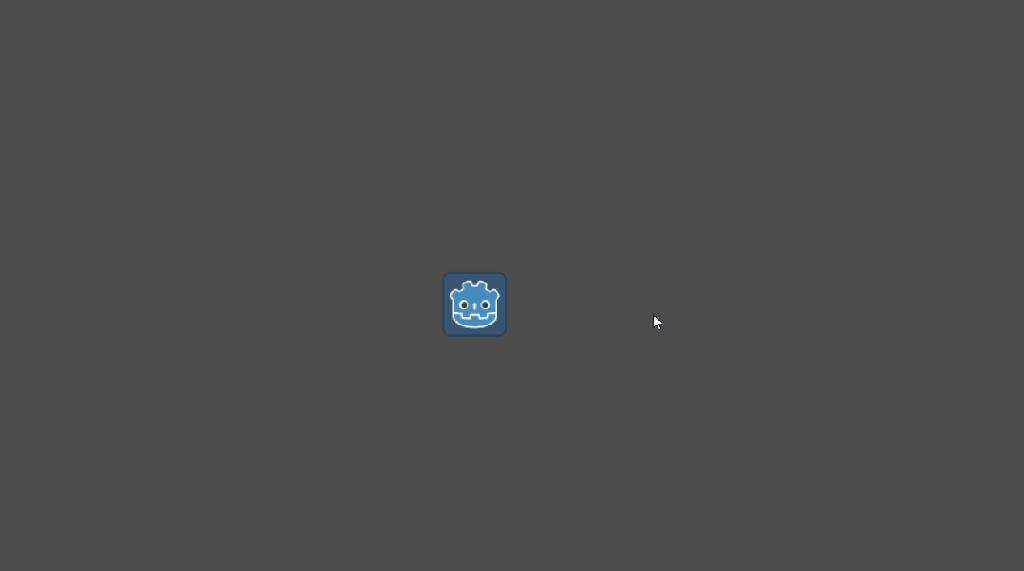
In the case of 2D we are going to take the input and add it to the position of the player node.
- Create a new script
- Copy and paste the code below into the script.
- If the actions you have mapped have different names, then change the name of the actions in the script.
extends Sprite
var speed = 20
func _process(delta):
if Input.is_action_pressed("ui_right"):
position.x+=20*speed*delta
if Input.is_action_pressed("ui_left"):
position.x-=20*speed*delta
if Input.is_action_pressed("ui_up"):
position.y-=20*speed*delta
if Input.is_action_pressed("ui_down"):
position.y+=20*speed*delta
Moving a Player in 3D
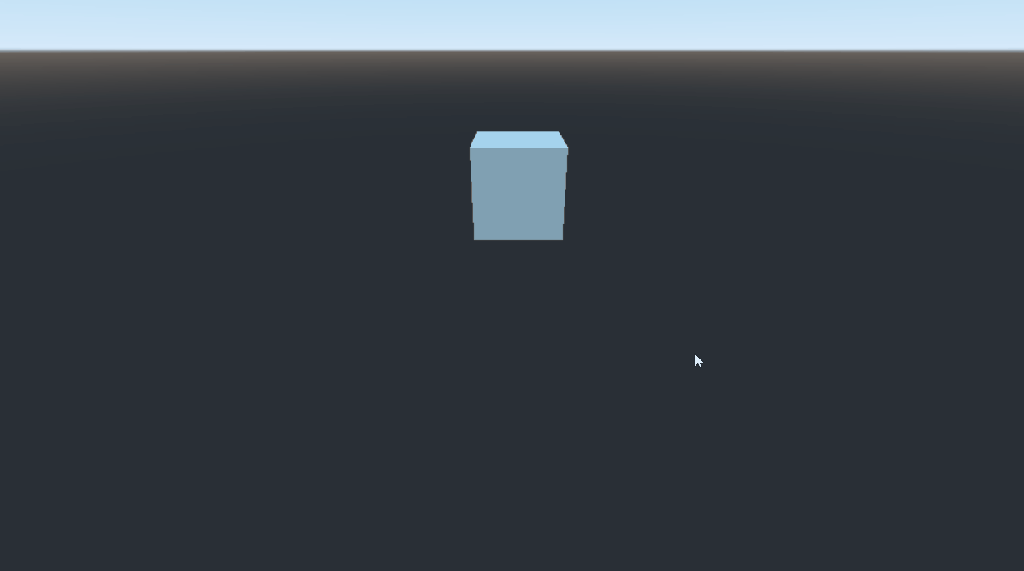
3D is same as 2D with a small difference. We take the up and down arrow input and add them to z axis in place of y axis as in case of 2D. Also position in 2D needs to be changed to translation in 3D.
Here is the final script that you can use to move an object in 3D in Godot.
extends Spatial
var speed=2
func _process(delta):
if Input.is_action_pressed("ui_right"):
translation.x+=2*speed*delta
if Input.is_action_pressed("ui_left"):
translation.x-=2*speed*delta
if Input.is_action_pressed("ui_up"):
translation.z-=2*speed*delta
if Input.is_action_pressed("ui_down"):
translation.z+=2*speed*delta
THANK YOU!
You have no idea how long it took me to find this!
One step closer to finally getting a game made!
Thank you so very much!
Glad it was helpful. Good luck with your game.