In this tutorial, we will see how to make a camera follow the player in Godot. I will be using Godot version 3.4.2 for this tutorial. If you use Unity, check out camera follow player Unity tutorial.
Camera follow player in Godot 2D.
- Right click on your scene and click add node. Seach for Camera2D and add it to the scene.
- Select the Camera2D node and go to the inspector window.
- Add a new script to camera and call it player_follow.
- Click the down arrow near the script and click edit.
- Copy and paste the code below in to the script.
- Save the script.
Camera follow script for 2D
extends Camera2D
var player
func _ready():
player= get_node("/root/Scene/Icon")
func _process(delta):
position.x=player.position.x
position.y=player.position.y
Save and run the game. The camera will follow the player movement in x and y axis.
Camera follow player in Godot 3D
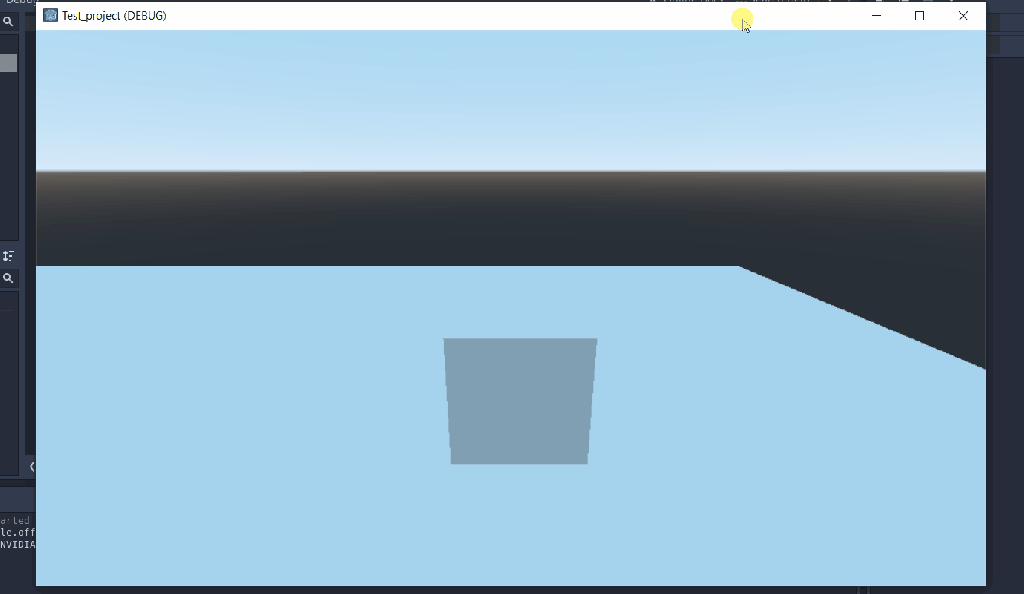
- Add Camera node in place of Camera2D
- Set your camera angle as required.
- In our case the player moves only in the x axis so let’s add the script for the camera to move only in the x axis.
- Add a script to the camera object.
- Copy and paste the code below.
- Save the code and run the project.
Camera follow script for 3D
extends Camera
# Declare member variables here. Examples:
# var a = 2
# var b = "text"
var player
# Called when the node enters the scene tree for the first time.
func _ready():
player= get_node("/root/Scene/glass cube")
# Called every frame. 'delta' is the elapsed time since the previous frame.
func _process(delta):
translation.x=player.translation.x
# pass